How do you declare a global variable in node JS?
To set up a global variable, we need to create it on the global object. The global object is what gives us the scope of the entire project, rather than just the file (module) the variable was created in. In the code block below, we create a global variable called globalString and we give it a value.
The global Keyword
Normally, when you create a variable inside a function, that variable is local, and can only be used inside that function. To create a global variable inside a function, you can use the global keyword.
The clean, reliable way to declare and define global variables is to use a header file to contain an extern declaration of the variable. The header is included by the one source file that defines the variable and by all the source files that reference the variable.
...
For example:
- function m(){
- window. value=100;//declaring global variable by window object.
- }
- function n(){
- alert(window. value);//accessing global variable from other function.
- }
Globals in Node.js - YouTube
Node. js Global Objects are the objects that are available in all modules. Global Objects are built-in objects that are part of the JavaScript and can be used directly in the application without importing any particular module.
A global variable is a variable that is declared in the global scope in other words, a variable that is visible from all other scopes. In JavaScript it is a property of the global object.
A global object is an object that always exists in the global scope. In JavaScript, there's always a global object defined. In a web browser, when scripts create global variables defined with the var keyword, they're created as members of the global object. (In Node. js this is not the case.)
Global variables are stored in the data section. Unlike the stack, the data region does not grow or shrink — storage space for globals persists for the entire run of the program.
The reason the first alert is undefined is because you re-declared global as a local variable below it in the function. And in javascript that means from the top of the function it is considered the local variable.
What are the problems with global variables in JavaScript?
This is because global variables are easily overwritten by other scripts. Global Variables are not bad and not even a security concern, but it shouldn't overwrite values of another variable. On the usage of more global variables in our code, it may lead to a maintenance issue.
Global variables can be accessed from anywhere in a JavaScript program. Variables declared with var , let and const are quite similar when declared outside a block.
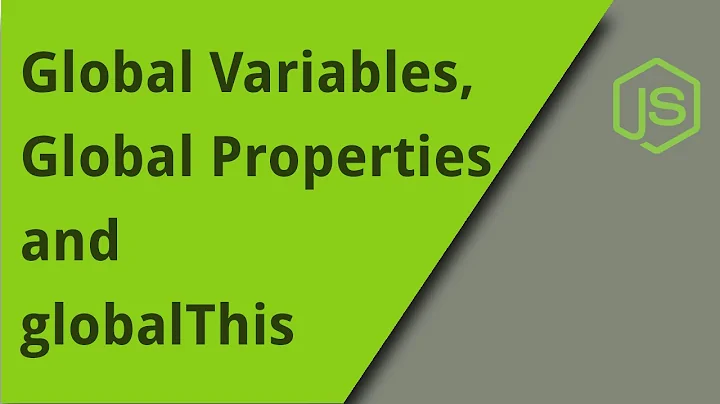
You can't define a global variable inside a function. You can implicitly create a global variable, or create a window property inside a function, but you can't define a global variable inside a function. With regards to JavaScript in a browser environment, a global variable and a property of window are synonymous.
So for your counter, make the global variable: var MYAPP = {}; and then the counter property MYAPP. counter = 0; . Then increment MYAPP. counter when appropriate.
Using global variables causes very tight coupling of code. Using global variables causes namespace pollution. This may lead to unnecessarily reassigning a global value. Testing in programs using global variables can be a huge pain as it is difficult to decouple them when testing.
Node. js global objects are global in nature and they are available in all modules. We do not need to include these objects in our application, rather we can use them directly. These objects are modules, functions, strings and object itself as explained below.
Install Package Globally
NPM installs global packages into /<User>/local/lib/node_modules folder. Apply -g in the install command to install package globally.
Are global variables stored in specific object? The answer is yes; they are stored in something called, officially, the global object. This object is described in Section 15.1 of the official ECMAScript 5 Specification.
Global variables should be used when multiple functions need to access the data or write to an object. For example, if you had to pass data or a reference to multiple functions such as a single log file, a connection pool, or a hardware reference that needs to be accessed across the application.
The globalBindings declaration has global scope and is used to customize the bindings globally for all schemas. A schema can have only one such declaration, and the declaration affects all the imported and included schemas.
What does global mean in coding?
A Global Variable in the program is a variable defined outside the subroutine or function. It has a global scope means it holds its value throughout the lifetime of the program. Hence, it can be accessed throughout the program by any function defined within the program, unless it is shadowed.
Avoid globals. Global variables and function names are an incredibly bad idea. The reason is that every JavaScript file included in the page runs in the same scope.
Variables stored in registers would need less power to access as there is no bus, address decoding and all that stuff you need for RAM access needed. Global variables will most likely always be stored in RAM if you don't do some crazy stuff with your compiler (allocating a register for a variable).
A global variable is a variable that is accessible globally. A local variable is one that is only accessible to the current scope, such as temporary variables used in a single function definition.
A static variable can be either a global or local variable. Both are created by preceding the variable declaration with the keyword static. A local static variable is a variable that can maintain its value from one function call to another and it will exist until the program ends.
You just use const at global scope: const aGlobalConstant = 42; That creates a global constant. It is not a property of the global object (because const , let , and class don't create properties on the global object), but it is a global constant accessible to all code running within that global environment.
A variable that has not been assigned a value is of type undefined . A method or statement also returns undefined if the variable that is being evaluated does not have an assigned value. A function returns undefined if a value was not returned .
The this keyword of the clickMe arrow function refers to the global object, in this case the window object. So, this. position and this. color will be undefined because our window object does not know anything about the position or the color properties.
In a scenario, where you need one central global point of access across the codebase, singletons are a good alternative for global variables. We can call a singleton as a lazily initialized global class which is useful when you have large objects — memory allocation can be deferred till when it's actually needed.
Non-const global variables are evil because their value can be changed by any function. Using global variables reduces the modularity and flexibility of the program. It is suggested not to use global variables in the program. Instead of using global variables, use local variables in the program.
Why are global variables a security risk?
Security is a function of understanding what your code does. Security, within a program, means "your code only does what it is intended to be allowed to do"; with global variables, you have a weaker ability to understand what the code does, thus less ability to control what it does.
Global Variables are the variables that can be accessed from anywhere in the program. These are the variables that are declared in the main body of the source code and outside all the functions. These variables are available to every function to access. Var keyword is used to declare variables globally.
A local variable is a variable defined within a function. Such variables are said to have local 'scope'. Functions can access global variables and modify them. Modifying global variables in a function is considered poor programming practice.
ES6 introduced the const keyword, which is used to define a new variable in JavaScript. Generally, the var keyword is used to declare a JavaScript variable. Const is another keyword to declare a variable when you do not want to change the value of that variable for the whole program.
Global Variables − A global variable has a global scope which means it can be defined anywhere in your JavaScript code. Local Variables − A local variable will be visible only within a function where it is defined. Function parameters are always local to that function.
- var global = "Global Variable"; //Define global variable outside of function.
-
- function setGlobal(){
- global = "Hello World!";
- };
- setGlobal();
- console. log(global); //This will print out "Hello World"
- About cookies on this site. ...
- public class CONSTANT { private CONSTANT() {} public static final integer SUCCESS = 1; public static final integer FAILURE = -1; public static final integer NOTFOUND = 0; } ...
- if (myMethod()==CONSTANT.SUCCESS) { ...; } else { ...; }
Create a file : import React from "react"; const AppContext = {}; export default AppContext; then in App. js, update the value import AppContext from './AppContext'; AppContext. username = uname. value; Now if you want the username to be used in another screen: import AppContext from './AppContext'; AppContext.
let allows you to declare variables that are limited in scope to the block, statement, or expression on which it is used. This is unlike the var keyword, which defines a variable globally, or locally to an entire function regardless of block scope.
To declare a global variable in TypeScript, create a . d. ts file and use declare global{} to extend the global object with typings for the necessary properties or methods.
How do you assign a value to a variable in NodeJS?
var getValue = function getValue(){ global. value = 5; }; console. log(value);
declare global is used inside a file that has import or export to declare things in the global scope. This is necessary in files that contain import or export since such files are considered modules, and anything declared in a module is in the module scope.
- Reference to global variable from a module.
- How to access JS global variable in Typescript file, using webpack.
- Use a variable from ancestor module when it's declared elsewhere.
- Using globally defined script inside the component.
- -1.
- Access JS created constant in TypeScript code.
If you create the array inside the function then it is only accessible from within the function body. You can declare it outside of the function: let ArrayRoute = []; function route(permutation, origins) { var myroute = origins[0]; ArrayRoute.
The type syntax for declaring a variable in TypeScript is to include a colon (:) after the variable name, followed by its type. Just as in JavaScript, we use the var keyword to declare a variable.
declare is used to tell the compiler "this thing (usually a variable) exists already, and therefore can be referenced by other code, also there is no need to compile this statement into any JavaScript". Common Use Case: You add a reference to your web page to a JavaScript file that the compiler knows nothing about.
Before you use a variable in a JavaScript program, you must declare it. Variables are declared with the var keyword as follows. Storing a value in a variable is called variable initialization. You can do variable initialization at the time of variable creation or at a later point in time when you need that variable.
ES6 introduced the const keyword, which is used to define a new variable in JavaScript. Generally, the var keyword is used to declare a JavaScript variable. Const is another keyword to declare a variable when you do not want to change the value of that variable for the whole program.
The simple assignment operator ( = ) is used to assign a value to a variable. The assignment operation evaluates to the assigned value.
declare class is for when you want to describe an existing class (usually a TypeScript class, but not always) that is going to be externally present (for example, you have two . ts files that compile to two . js files and both are included via script tags in a webpage).
How do I export a variable in TypeScript?
Use named exports to export multiple variables in TypeScript, e.g. export const A = 'a' and export const B = 'b' . The exported variables can be imported by using a named import as import {A, B} from './another-file' . You can have as many named exports as necessary in a single file.